Details
-
AboutI’m full-stack web-mobile-developer with 11+ years of experience.
-
Skillsjavascript, react, react-native, go, python
-
LocationNetherlands
-
Website
-
Github
Joined devRant on 5/22/2018
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
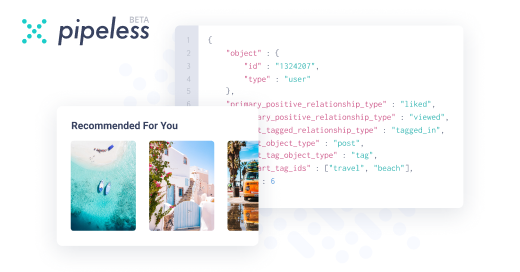
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
-
I was curious about the geographical distribution of Top Tech Jobs (i.e. how many FAANG jobs are in Europe, where they are mostly focused, etc)... So I came up with a draft (prototype) of the Tech Jobs Radar:
https://jobs-radar.com/
I'm sharing it here just in case someone else is curious about analyzing such data7 -
For learning purposes, I made a minimal TensorFlow.js re-implementation of Karpathy’s minGPT (Generative Pre-trained Transformer). One nice side effect of having the 300-lines-of-code model in a .ts file is that you can train it on a GPU in the browser.
https://github.com/trekhleb/...
The Python and Pytorch version still seems much more elegant and easy to read though...6 -
I recently went through a very detailed and well-explained Python-based project/lesson by Karpathy which is called micrograd. This is a tiny scalar-valued autograd engine and a neural net on top of it.
The project above is, as expected, built on Python. For learning purposes, I wanted to see how such a network may be implemented in TypeScript and came up with a 🤖 micrograd-ts - https://github.com/trekhleb/... repository (and also with a demo - https://trekhleb.dev/micrograd-ts/ of how the network may be trained).
Trying to build anything on your own very often gives you a much better understanding of a topic. So, this was a good exercise, especially taking into account that the whole code is just ~200 lines of TS code with no external dependencies.
The micrograd-ts repository might be useful for those who want to get a basic understanding of how neural networks work, using a TypeScript environment for experimentation.
With that being said, let me give you some more information about the project.
## Project structure
- [micrograd/](https://github.com/trekhleb/...) — this folder is the core/purpose of the repo
- [engine.ts](https://github.com/trekhleb/...) — the scalar `Value` class that supports basic math operations like `add`, `sub`, `div`, `mul`, `pow`, `exp`, `tanh` and has a `backward()` method that calculates a derivative of the expression, which is required for back-propagation flow.
- [nn.ts](https://github.com/trekhleb/...) — the `Neuron`, `Layer`, and `MLP` (multi-layer perceptron) classes that implement a neural network on top of the differentiable scalar `Values`.
- [demo/](https://github.com/trekhleb/...) - demo React application to experiment with the micrograd code
- [src/demos/](https://github.com/trekhleb/...) - several playgrounds where you can experiment with the `Neuron`, `Layer`, and `MLP` classes.
Demo (online)
---------------------
To see the online demo/playground, check the following link:
🔗 https://trekhleb.dev/micrograd-ts3 -
I've played around a bit with illustrating the SOLID principles (Single Responsibility, Open/Closed, etc., you know).
You may check it out here: https://okso.app/showcase/solid
Let's see if it will be helpful or more confusing :D13 -
Recently I launched the minimalistic online drawing app https://okso.app. I wanted it to be a place where people could do fast, ad-hoc, napkin-based-like explanations of any concept as if you are sitting with your friend and trying to explain him/her something during lunch. Don't ask me why it is needed, I was just experimenting.
So, the first concept I've tried to explain with sketches was the Data Structures. Without further ado, here is the interactive ✍🏻 https://okso.app/showcase/... showcase that you may play with.
Of course, not all data structures are covered. And of course, this is not comprehensive material, but rather a cheatsheet that would create visual hints and associations for the following data structures:
- Linked List
- Doubly Linked List
- Queue
- Stack
- Hash Table (with hash collision resolution)
- Tree (including the Binary Search Tree)
- Heap (including Mean Heap and Max Heap)
- Trie
- Graph
Each box on the sketch is clickable, so you may dig into the data structure you're interested. For example `Heap → Max Heap`, or `Heap → Min Heap`, or `Heap → Array Representation`.
The sketches are split into so-called Pages just to make it easier to grasp them, so the users stay focused on one concept at a time, they see the relationship between the concept, and thus, hopefully, they are not getting overwhelmed with seeing a lot of information at the same time on one drawing/page.
Each page has a link to the source-code examples that are implementing the data structure on JavaScript.
The full list you may find in the ✍🏻 https://okso.app/showcase/... showcase.
I hope you find this showcase useful and I hope it will be a good visual cheatsheet-like complement to your data structure knowledge.12 -
Hey folks, I've just launched the https://okso.app - it is a drawing app that you may use to express, grasp, and organize your thoughts and ideas.
One key feature there is that you may organize your drawings/sketches into a hierarchical tree structure so that a large amount of data would be more manageable and less overwhelming.
I hope you find this app useful!10 -
I was curious about how the Genetic Algorithm works, wanted to try it out.
So I've created some toy cars using Three.js and "asked" them to do the self-parking with a little bit of Genetic Algorithm help.
It was fun to see how those toy cars were evolving and actually started to be less stupid :D
Here are some more details:
https://trekhleb.dev/blog/2021/...10 -
I was working on a project lately where I needed to convert an array of bits (1s and 0s) to floating numbers.
It is quite straightforward how to convert an array of bits to the simple integer (i.e. [101] = 5). But what about the numbers like `3.1415` (𝝿) or `9.109 × 10⁻³¹` (the mass of the electron in kg)?
I've explored this question a bit and came up with a diagram that explains how the float numbers are actually stored.
There is also an interactive version of this diagram available here https://trekhleb.dev/blog/2021/....
Feel free to experiment with it and play around with setting the bits on and off and see how it influences the final result.13 -
Recently I've played around with the Seam Carving algorithm for the content-aware image resizing.
It turned out that the algorithm is pretty powerful, elegant but yet simple. One of the interesting parts was that it might be optimized with the Dynamic Progrming approach. Also, it may perform a simple object removal from the image without even modern ML algorithms.
I've tried to describe my experience here in the interactive article:
https://trekhleb.dev/blog/2021/...4 -
Dynamic Programming vs Divide-and-Conquer
👉🏻 https://trekhleb.dev/blog/2018/...
In this article, I’m trying to figure out the difference/similarities between dynamic programming and divide and conquer approaches based on two examples - binary search and minimum edit distance (Levenshtein distance).
The DP concept is still a subject to learn for me, but I hope the article will be helpful for those who are also in the process of learning. -
I read a book with printed links in it, but I couldn’t click on them 🤷🏻♂️
So I made an alpha version of the 📖👈🏻 Links Detector app that makes printed links clickable.
Check it out 👉🏻 https://trekhleb.github.io/links-de...30 -
Hello Readers!
I’ve recently launched JavaScript Algorithms and Data Structures repository on GitHub with collection of classic algorithms and data-structures implemented in ES6 JavaScript with explanations and links to further readings and YouTube videos.
You may find it here https://github.com/trekhleb/...
So I guess you’ve already grasp main idea of the project — helping developers to learn and practice algorithms and do it in JavaScript.
To make this process even smoother I’ve tried to put some graphical illustrations for each algorithm and data structure where it was possible just to make the idea behind those algorithms to be easily grasped and memorized.
You also may find some practical information just in the root README file that may be handy while you’re studying.
All code is 100% covered with tests. This is done not only to keep code working correctly but also to give you an illustration of how each algorithms or data structure works, what basic operations they have (let’s say polling for heap) and what are the edge cases (what to do if graph is directed).
Repository also has a playground. This is just small function template along with empty test case that will help you to start testing or working on algorithms just right after cloning the repo.
On top of that data structures there are more then 50 popular algorithms are implemented. Among them are sorting, searching algorithms, graph/tree/sets/string/math related algorithms.
I hope this repository will be helpful for you! Enjoy coding!2